Traffic Sign Detection Project Using Resnet
INTRODUCTION:
Residual Networks, or ResNets, learn residual functions with reference to the layer inputs, instead of learning unreferenced functions. Instead of hoping each few stacked layers directly fit a desired underlying mapping, residual nets let these layers fit a residual mapping.
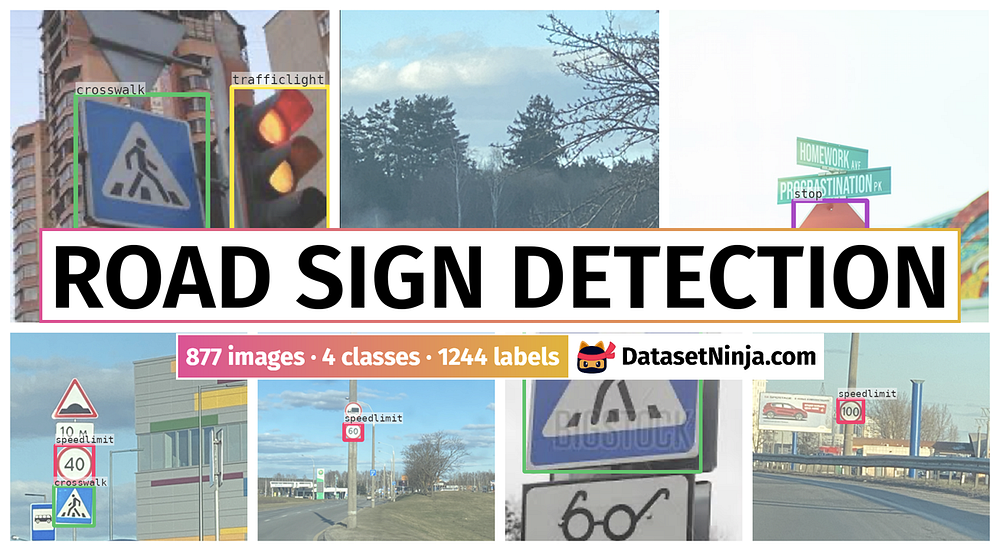
CODE ๐๐:
from fastai.vision.all import *
import numpy as np
import pandas as pd
from pathlib import Path
path = Path(โpath/to/your/traffic-signsโ)
np.random.seed(42)
data_block = DataBlock(
blocks=(ImageBlock, CategoryBlock), # Define image classification task
get_items=get_image_files, # Get all image files
splitter=RandomSplitter(valid_pct=0.2, seed=42), # 20% data for validation
get_y=parent_label, # Labels are the parent directory names
item_tfms=Resize(224), # Resize images to 224x224 for ResNet50
batch_tfms=aug_transforms(mult=1.0) # Apply data augmentation
)
dls = data_block.dataloaders(path, bs=64)
# Batch size of 64
dls.show_batch(max_n=9, figsize=(8, 8))
learn = cnn_learner(dls, resnet50, metrics=[accuracy])
learn.fine_tune(5) # Fine-tune the model for 5 epochs
img_path = โpath/to/your/test-image.jpgโ
img = PILImage.create(img_path)
pred_class, pred_idx, outputs = learn.predict(img)
print(fโPredicted class: {pred_class}โ)
learn.export(โtraffic_sign_resnet50_model.pklโ)
No comments:
Post a Comment