INTRODUCTION:
SQL injection, also known as SQLI, is a common attack vector that uses malicious SQL code for backend database manipulation to access information that was not intended to be displayed. This information may include any number of items, including sensitive company data, user lists or private customer details.
The impact SQL injection can have on a business is far-reaching. A successful attack may result in the unauthorized viewing of user lists, the deletion of entire tables and, in certain cases, the attacker gaining administrative rights to a database, all of which are highly detrimental to a business.
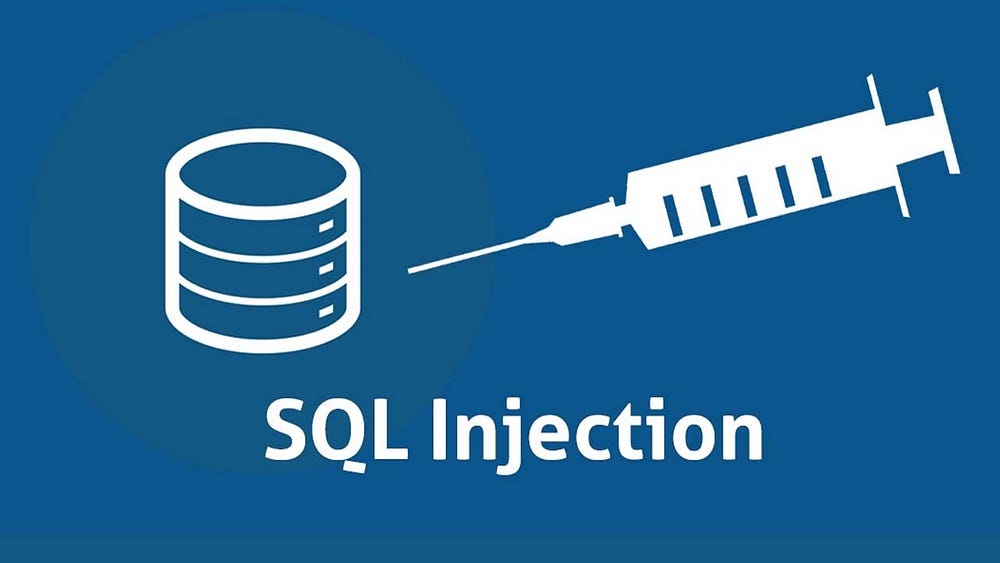
CODE 😃👇:
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score, classification_report
import pickle
# Load data
data = pd.read_csv(‘sql_injection_data.csv’)
# Check for missing values and clean if necessary
data.dropna(inplace=True)
# Split dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(
data[‘query’], data[‘label’], test_size=0.2, random_state=42)
# Transform queries using TF-IDF
vectorizer = TfidfVectorizer(max_features=1000)
X_train_tfidf = vectorizer.fit_transform(X_train)
X_test_tfidf = vectorizer.transform(X_test)
# Initialize and train the classifier
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train_tfidf, y_train)
# Evaluate on the test set
y_pred = model.predict(X_test_tfidf)
print(“Accuracy:”, accuracy_score(y_test, y_pred))
print(“Classification Report:\n”, classification_report(y_test, y_pred))
# Save model
with open(‘sql_injection_model.pkl’, ‘wb’) as model_file:
pickle.dump(model, model_file)
# Save vectorizer
with open(‘tfidf_vectorizer.pkl’, ‘wb’) as vectorizer_file:
pickle.dump(vectorizer, vectorizer_file)
def detect_sql_injection(query):
# Load model and vectorizer
with open(‘sql_injection_model.pkl’, ‘rb’) as model_file:
loaded_model = pickle.load(model_file)
with open(‘tfidf_vectorizer.pkl’, ‘rb’) as vectorizer_file:
loaded_vectorizer = pickle.load(vectorizer_file)
# Transform the query
query_tfidf = loaded_vectorizer.transform([query])
# Predict
prediction = loaded_model.predict(query_tfidf)
return “Malicious” if prediction[0] == 1 else “Benign”
# Test with a sample input
print(detect_sql_injection(“SELECT * FROM users WHERE id=1”)) # Expected output: “Benign” or “Malicious”
DATASET 😃👇
https://www.kaggle.com/datasets/syedsaqlainhussain/sql-injection-dataset
SUPPORT ME 😟
FREE C++ SKILLSHARE COURSE
FREE C SKILLSHARE COURSE
All Courses 😃👇
https://linktr.ee/Freetech2024
All Products 😃👇
https://linktr.ee/rockstararun
HP Laptop 🤩👇
Asus Laptop 🤩👇
No comments:
Post a Comment