Encryption & Decryption Using Fernet Symmetric Method
INTRODUCTION:
Fernet is a symmetric encryption method, meaning the same key is used for both encryption and decryption. It ensures that your data is secure while also being easy to implement and use. Fernet guarantees the following properties: Confidentiality: Only authorized parties can read the encrypted data
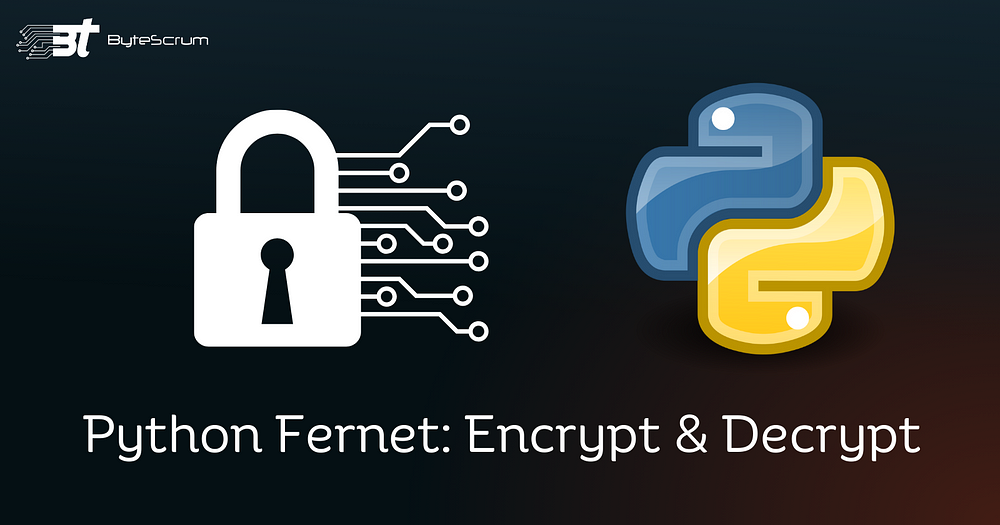
CODE 😃👇:
pip install cryptography
from cryptography.fernet import Fernet
import os
# Function to generate and save the encryption key
def generate_key(key_filename=”encryption.key”):
key = Fernet.generate_key()
with open(key_filename, “wb”) as key_file:
key_file.write(key)
print(f”Key saved to {key_filename}”)
# Function to load the encryption key
def load_key(key_filename=”encryption.key”):
return open(key_filename, “rb”).read()
# Function to encrypt a file
def encrypt_file(input_file, key_filename=”encryption.key”):
# Load the key
key = load_key(key_filename)
fernet = Fernet(key)
# Read the file content
with open(input_file, “rb”) as file:
file_data = file.read()
# Encrypt the file data
encrypted_data = fernet.encrypt(file_data)
# Save the encrypted data back to the file
encrypted_file = input_file + “.encrypted”
with open(encrypted_file, “wb”) as file:
file.write(encrypted_data)
print(f”File ‘{input_file}’ encrypted to ‘{encrypted_file}’”)
# Function to decrypt an encrypted file
def decrypt_file(encrypted_file, key_filename=”encryption.key”):
# Load the key
key = load_key(key_filename)
fernet = Fernet(key)
# Read the encrypted file data
with open(encrypted_file, “rb”) as file:
encrypted_data = file.read()
# Decrypt the file data
try:
decrypted_data = fernet.decrypt(encrypted_data)
decrypted_file = encrypted_file.replace(“.encrypted”, “.decrypted”)
# Save the decrypted data back to a file
with open(decrypted_file, “wb”) as file:
file.write(decrypted_data)
print(f”File ‘{encrypted_file}’ decrypted to ‘{decrypted_file}’”)
except Exception as e:
print(f”Decryption failed: {e}”)
# Main code for running the tool
def main():
print(“File Encryption and Decryption Tool”)
print(“1. Generate Encryption Key”)
print(“2. Encrypt File”)
print(“3. Decrypt File”)
choice = input(“Choose an option (1/2/3): “)
if choice == “1”:
key_filename = input(“Enter key filename (default: encryption.key): “) or “encryption.key”
generate_key(key_filename)
elif choice == “2”:
input_file = input(“Enter the path of the file to encrypt: “)
key_filename = input(“Enter key filename (default: encryption.key): “) or “encryption.key”
encrypt_file(input_file, key_filename)
elif choice == “3”:
encrypted_file = input(“Enter the path of the file to decrypt: “)
key_filename = input(“Enter key filename (default: encryption.key): “) or “encryption.key”
decrypt_file(encrypted_file, key_filename)
else:
print(“Invalid choice. Please try again.”)
if name == “main”:
main()
No comments:
Post a Comment