Emotion Detection From Text Using NLP
INTRODUCTION :
Natural language processing helps computers communicate with humans in their own language and scales other language-related tasks. For example, NLP makes it possible for computers to read text, hear speech, interpret it, measure sentiment and determine which parts are important.
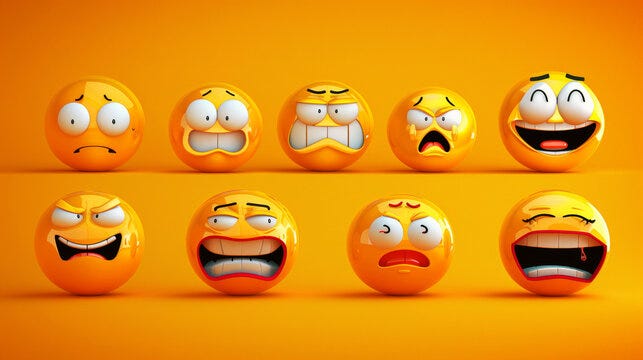
CODE 😃👇 :
import nltk
from sklearn.model_selection import train_test_split
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.naive_bayes import MultinomialNB
from sklearn.metrics import accuracy_score, classification_report
from transformers import pipeline
import pandas as pd
# Download necessary NLTK data
nltk.download(‘stopwords’)
# Step 1: Data Collection (Using the Text Emotion Dataset or Custom Dataset)
# Sample dataset (you can replace it with a larger dataset like Text Emotion Dataset)
data = {
‘text’: [
“I am so happy today!”,
“I am feeling very sad.”,
“What a beautiful day!”,
“I am angry with you!”,
“I feel so surprised and amazed.”,
“I hate waiting in lines.”,
“This is awesome!”,
“I don’t know what to do, I feel lost.”,
],
‘emotion’: [‘joy’, ‘sadness’, ‘joy’, ‘anger’, ‘surprise’, ‘anger’, ‘joy’, ‘fear’]
}
df = pd.DataFrame(data)
# Step 2: Text Preprocessing (Cleaning)
def preprocess_text(text):
# Convert to lowercase, remove punctuation and stopwords
text = text.lower()
return text
df[‘text’] = df[‘text’].apply(preprocess_text)
# Step 3: Feature Extraction using TF-IDF
X = df[‘text’]
y = df[‘emotion’]
# Split data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# TF-IDF Vectorizer to convert text into features
vectorizer = TfidfVectorizer()
X_train_tfidf = vectorizer.fit_transform(X_train)
X_test_tfidf = vectorizer.transform(X_test)
# Step 4: Train a Classifier
# Using Naive Bayes Classifier
classifier = MultinomialNB()
classifier.fit(X_train_tfidf, y_train)
# Step 5: Predictions and Evaluation
y_pred = classifier.predict(X_test_tfidf)
# Print accuracy and classification report
print(f”Accuracy: {accuracy_score(y_test, y_pred):.2f}”)
print(“Classification Report:\n”, classification_report(y_test, y_pred))
# Step 6: Emotion Detection using Hugging Face Transformers (Pre-trained Emotion Detection Model)
# You can also use a pre-trained model for emotion detection like ‘j-hartmann/emotion-english-distilroberta-base’ from Hugging Face
emotion_pipeline = pipeline(“text-classification”, model=”j-hartmann/emotion-english-distilroberta-base”)
# Test the model with a sample text
sample_text = “I feel so amazing and excited!”
emotion = emotion_pipeline(sample_text)
print(f”Detected Emotion: {emotion[0][‘label’]}, with score: {emotion[0][‘score’]:.2f}”)
No comments:
Post a Comment